How to remove old feature flags without breaking your code
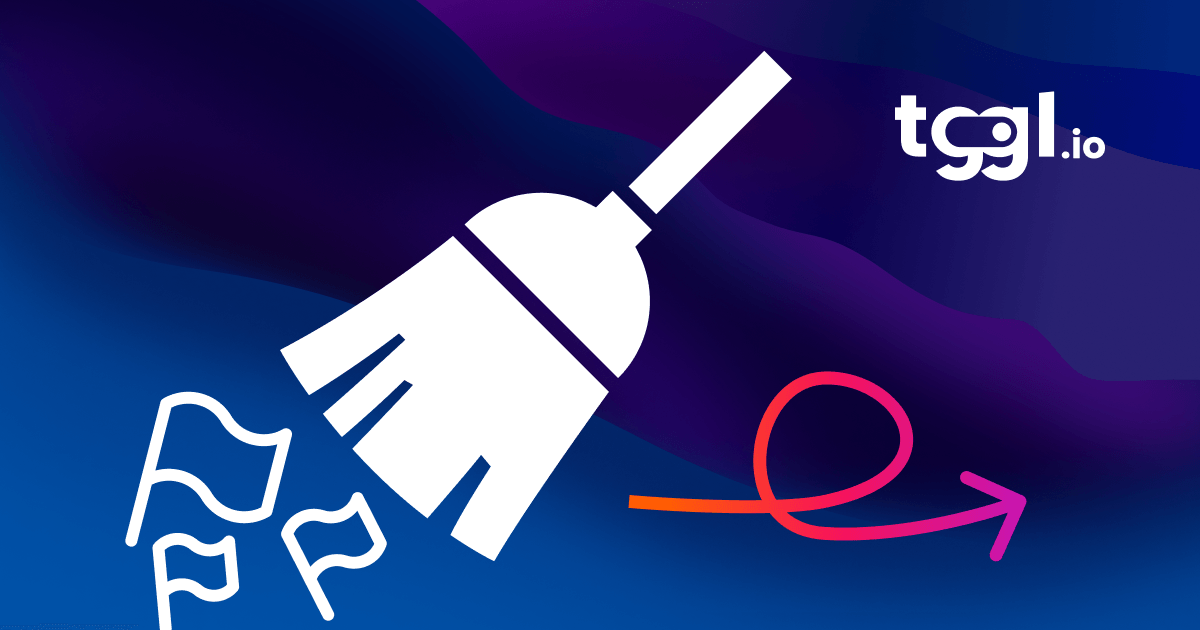
Feature flags are a game-changer for releasing features safely and giving teams more control over deployments. But there’s a catch, if you don’t clean up old feature flags, they start piling up and creating problems. Over time, they can slow down development, add unnecessary complexity, and even introduce security risks.
Teams often move fast when adding new flags but forget to remove them once a feature is fully rolled out. When old flags linger in the codebase, they make it harder to debug, increase cognitive load for developers, and can even lead to unexpected behavior in production.
So how do you know when it’s time to remove a feature flag? And more importantly, how do you clean up old flags without accidentally breaking production?
In this guide, we’ll break it down step by step:
- How to identify stale feature flags
- Best practices for removing them safely
- How to automate flag cleanup so your codebase stays clean
The risks of leaving old feature flags in your code
Adding feature flags is easy. Removing them? That’s where teams often get stuck. When old flags stay in the codebase long after they’ve served their purpose, they create technical debt that slows development and increases risk. Here’s what happens when feature flags aren’t properly cleaned up:
1. Slower development & more complexity
Every feature flag adds a conditional statement to your code. When flags pile up, developers have to navigate unnecessary branches every time they make changes. This leads to:
- More time spent debugging (“Is this flag still active?”)
- Higher cognitive load for developers trying to understand the codebase
- Increased risk of errors from outdated or forgotten flags
2. Harder debugging & unexpected behavior
Old flags create confusion. If a bug appears and no one knows whether a flag is still in use, it slows down troubleshooting. Even worse, some flags might still be toggling behavior in production without anyone realizing it.
3. Security risks
Feature flags control access to certain functionalities. If old flags are left in the code:
- Deprecated features could accidentally be reactivated.
- Access controls might not work as intended.
- Sensitive data exposure becomes a risk if flags aren’t properly managed.
4. Performance impact
While a single flag doesn’t significantly affect performance, a large number of outdated flags can:
- Increase execution time due to unnecessary condition checks.
- Bloat database queries if flags are stored and checked dynamically.
- Cause unexpected slowdowns in production environments.
The bottom line? Unused feature flags aren’t just harmless leftovers, they actively make your codebase harder to maintain, less secure, and more prone to bugs.
In the next section, we’ll go over how to identify which feature flags need to go and which ones are still useful.
How to identify stale feature flags
Before removing a feature flag, you need to be sure it’s no longer needed. The problem? Many teams don’t track their flags properly, so it’s not always obvious which ones are safe to delete. Here’s how to identify stale feature flags without risking production issues.
1. Audit your feature flags
Start by listing all active flags in your system. If you’re using a feature flagging tool like Tggl, this is easy, just check the dashboard to see which flags are in use.
Look for flags that:
- Haven’t been toggled in months
- Were created for one-time rollouts but never removed
- No longer align with any active features or experiments
2. Check code references
Some flags may still exist in the code but aren’t actually used anymore. To find them:
- Run a search in your codebase (
grep
,find
, or IDE search tools) - Use static analysis tools to detect dead code paths
- Check feature flag logs to see when the flag was last toggled
If a flag is referenced but never changes state, it’s a strong sign that it’s no longer needed.
3. Review flag ownership
Every feature flag should have a clear owner, someone responsible for deciding when to retire it. If no one knows what a flag does or why it exists, it’s probably safe to remove.
In Tggl, you can assign owners to feature flags, making it easier to track who’s responsible for cleanup.
4. Look for flags that were meant to be temporary
Some feature flags are meant to stay in place permanently (e.g., kill switches, permission-based flags). Others were only needed for:
- Gradual rollouts
- A/B testing
- Hotfixes and emergency patches
If the original purpose of a flag is no longer relevant, it should be removed.
What’s next? Now that you’ve identified stale feature flags, it’s time to remove them safely. In the next section, we’ll walk through best practices to delete old flags without breaking production.
Best practices for removing old feature flags
Now that you’ve identified which feature flags are stale, the next step is removing them safely. Deleting a flag without proper checks can introduce bugs, regressions, or unexpected behavior in production, so it’s important to follow a structured approach.
Here’s how to remove feature flags without breaking anything.
Step 1: Disable the flag in production
Before deleting any flag, first turn it off for all users. This ensures that the code using the flag runs as if the flag never existed.
- In Tggl: Simply toggle the flag off and monitor for any unexpected issues.
- For database-stored flags: Set the default value to "off" and let it run for a few days.
Monitor logs, error reports, and performance metrics to make sure nothing breaks.
Step 2: Remove flag references from your codebase
Once you’re confident that disabling the flag hasn’t caused issues, it’s time to remove it from the code.
- Search for all instances of the flag in your codebase using IDE search tools,
grep
, or static analysis tools. - Delete conditional statements that reference the flag (
if flag_enabled then ... else ...
). - Refactor affected code to ensure it functions correctly without the flag.
If your team uses feature flag lifecycle tracking, mark the flag as “deprecated” before removing it entirely.
Step 3: Clean up configuration and documentation
Even after removing the flag from the codebase, there may be lingering references in:
- Feature flag dashboards (like Tggl)
- Database tables storing flag states
- Internal documentation or Jira tickets
Make sure to delete outdated references so your team doesn’t waste time wondering if the flag still exists.
Step 4: Scale flag cleanup for the future
Manually tracking and removing feature flags isn’t scalable. Instead, set up processes that make it easier:
- Use expiration dates: In Tggl, you can set reminders or auto-expiry for temporary flags.
- Assign flag owners: Ensure every flag has a responsible team member to review its lifecycle.
- Monitor flag usage: Regularly audit feature flags to prevent unnecessary accumulation.
The bottom line? Most feature flags are meant to be temporary, and keeping old ones around only slows your team down. By following a structured removal process, you can clean up your codebase without breaking production.
In the next section, we’ll look at when it actually makes sense to keep a feature flag in place.
When should you keep a feature flag?
Not all feature flags should be removed. While many flags are temporary and meant to be cleaned up, some serve a long-term purpose and should stay in place. Here are a few cases where keeping a feature flag makes sense:
1. Kill switches for critical features
A kill switch is a permanent feature flag that allows you to instantly disable a feature if something goes wrong in production.
Use case: If a feature relies on a third-party API, a kill switch lets you turn it off if the API fails, without needing a full redeployment.
Keep kill switches clearly documented so engineers know they exist when troubleshooting incidents.
2. Regional or compliance-based rollouts
Some features need to be enabled only in certain locations or for specific users due to legal or business requirements.
Use case: If a payment method is only available in Europe, you can control access with a feature flag instead of hardcoding logic.
Use role-based access controls to ensure the right teams manage these flags.
3. Long-term A/B testing or feature experiments
While most A/B tests are temporary, some teams run ongoing experiments to fine-tune product performance.
Use case: If you're continuously adjusting an algorithm (e.g., recommendation engines, pricing models), a feature flag lets you tweak parameters without shipping new code.
Regularly review these flags and consolidate experiments to avoid clutter.
4. Permission-based feature access
Some teams use feature flags to manage internal tools or user access levels instead of using a full permissions system.
Use case: Allowing users to access a feature depending on their subscribed plan.
If a feature flag is meant to act as a long-term permission toggle, consider integrating it into your authentication system.
Final check: should this flag stay or go?
Before deciding to keep a feature flag, ask yourself:
- Does this flag still serve an active purpose?
- Is it documented, or would a new developer be confused about why it exists?
- Would it be better handled through config management or permissions instead of a flag?
If a flag isn’t actively serving a function, it’s probably time to clean it up.
Conclusion:
Feature flags give teams flexibility, faster releases, and safer deployments, but only if they’re managed properly. Leaving old flags in your codebase creates unnecessary complexity, slows development, and can even introduce security risks.
By following the right cleanup process, you can keep your feature flag system organized without breaking production:
- Regularly audit your feature flags to identify which ones are no longer needed.
- Disable stale flags first before removing them from your codebase.
- Clean up related configurations and documentation to prevent confusion.
- Use automation (like expiration dates and monitoring tools) to prevent flag bloat in the future.
- Only keep flags that serve a long-term purpose, such as kill switches or permission-based access.
The key takeaway? Feature flags should be an asset, not a liability. A well-maintained flag system keeps your codebase clean and your releases smooth.
If you want to manage feature flags the right way, Tggl makes it easy to track, monitor, and remove flags when they’re no longer needed.
Start using Tggl today and take control of your feature flag lifecycle.