.NET
Installation
Install the Tggl
package:
dotnet add package Tggl
Quick start
Instantiate a client with your API key and call EvalContext
to evaluate a context. A single API call evaluating all flags is performed, making all subsequent flag checking methods extremely fast.
This means that you do not need to cache results of GetX
methods since they do not trigger an API call, they simply look up the data in the already fetched response.
using Tggl;
var client = new TgglClient("YOUR_API_KEY");
var flags = await client.EvalContextAsync(new
{
UserId = "foo",
Email = "foo@gmail.com"
});
if (flags.GetString("my-feature", "Variation A") == "Variation A")
{
Console.WriteLine("Feature uses Variation A");
}
Different value types
You can get the value of a flag as a boolean, string, integer, double, list, or dictionary:
flags.GetBoolean("feat", true);
flags.GetString("feat", "foo");
flags.GetInteger("feat", 42);
flags.GetDouble("feat", 3.14);
flags.GetList("feat", []);
flags.GetDictionary("feat", new Dictionary<string, object?>());
Those methods are guaranteed to return the expected type. The default value is returned if the flag does not exist (or in case of network errors) or if its value is not of the expected type.
Alternatively you can call Get
which does not perform any type conversion:
flags.Get("feat", "foo");
Get
will always return the value of the flag if it exists, even if that value is null
, and will return the default value otherwise.
The context object
The flag evaluation context can be either a specific class, an anonymous object, or a Dictionary.
await client.EvalContextAsync(new
{
UserId = "foo",
Email = "foo@gmail.com"
});
await client.EvalContextAsync(new CustomContextClass
{
UserId = "foo",
Email = "foo@gmail.com"
});
await client.EvalContextAsync(new Dictionary<string, object>
{
{ "UserId", "foo" },
{ "Email", "foo@gmail.com" }
});
Evaluating contexts in batches
If you have multiple contexts to evaluate at once, you can batch your calls in a single HTTP request which is much more performant:
var result = await client.EvalContextsAsync([
new { UserId = "foo" },
new { UserId = "bar" }
]);
// Responses are returned in the same order
var fooFlags = result[0];
var barFlags = result[1];
Reporting
By default, the SDK sends flag evaluation data to Tggl to give you precious insights on how you are actually using your flags in production and to help you manage flags lifecycles better.
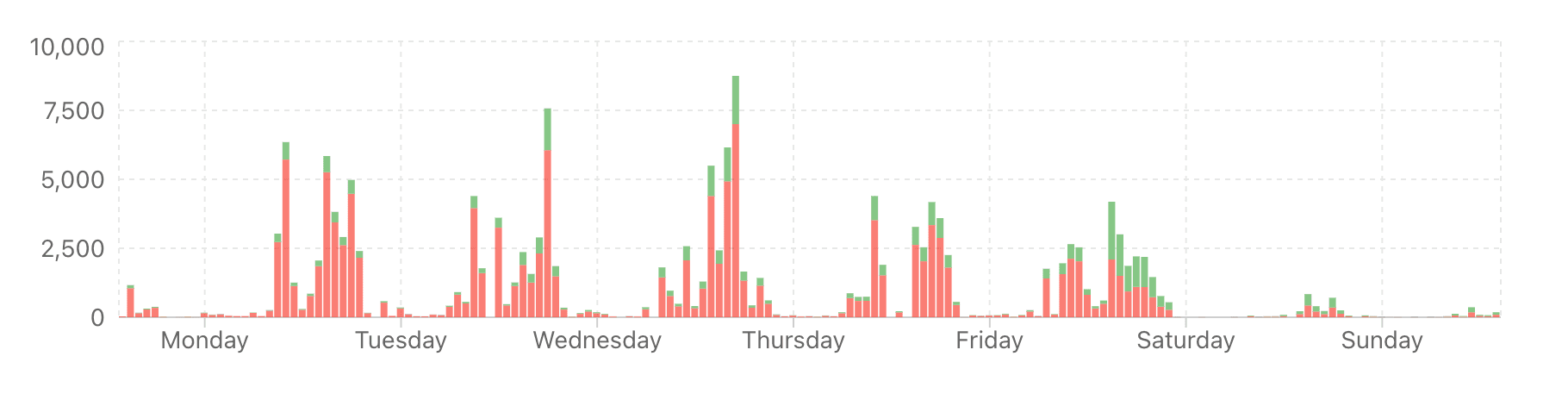
The SDK sends at most one HTTP request every 5 seconds to Tggl. Additionally, you can identify the client making the request by giving it an app name. You will be able to retrieve that name on the Tggl dashboard:
var client = new TgglClient("API_KEY", new TgglClient.Options
{
Reporting = new TgglReporting.Options
{
App = "My App:2.16.3"
},
});
You can also disable reporting entirely:
// Disable reporting when you instantiate the client
var client = new TgglClient("API_KEY", new TgglClient.Options
{
Reporting = false,
});
// Or disable it only for one response
var client = new TgglClient("API_KEY");
var flags = await client.EvalContextAsync(/*...*/)
flags.DisableReporting()
Using the Proxy
By default, the SDK talks directly to the Tggl API. If you are using the Tggl Proxy, you can specify the proxy URL when instantiating the client:
var client = new TgglClient("API_KEY", new TgglClient.Options
{
BaseUrl = "http://your-proxy-domain.com"
});
The /flags
and /report
path will be appended to the BaseUrl
and both flags evaluation and reporting will go through the proxy. If your proxy is configured with custom paths, you can specify them:
var client = new TgglClient("API_KEY", new TgglClient.Options
{
Url = "http://your-proxy-domain.com/custom-flags",
Reporting = new TgglReporting.Options
{
Url = "http://your-proxy-domain.com/custom-report"
},
});
Evaluate flags locally
It is possible to evaluate flags locally on the server but not recommended unless you have performance issues evaluating flags at a high frequency. Evaluating flags locally forces you to maintain the copy of flags configuration up to date and might be a source of issues.
Make sure to add the right keys to your context to be perfectly consistent with the Tggl API.
using Tggl;
var client = new TgglLocalClient("YOUR_SERVER_API_KEY");
// Wait for the config to be fetched
await client.Ready();
var context = new
{
UserId = "foo",
Email = "foo@gmail.com"
};
// Evaluation is performed locally
if (client.GetString(context, "my-feature", "Variation A") == "Variation A")
{
Console.WriteLine("Feature uses Variation A");
}
The config will be fetched every 5 seconds by default. You can change this interval by passing a PollingInterval
parameter to the constructor.
new TgglLocalClient("API_KEY", new TgglLocalClient.Options
{
PollingInterval = 10_000
});
Note that client.Ready()
will return immediately if the config has already been fetched, it only waits for the first initial config.