.NET
Installation
Add the OpenFeature
SDK and the Tggl.OpenFeature
provider to your project:
dotnet add package OpenFeature Tggl.OpenFeature
Quick start
Set the provider with your API key and create a client:
using OpenFeature;
using Tggl.OpenFeature;
await Api.Instance.SetProviderAsync(new TgglProvider("YOUR_API_KEY"));
var client = Api.Instance.GetClient();
var value = await client.GetStringValueAsync("my-feature", "Variation A");
if (value == "Variation A")
{
Console.WriteLine("Feature uses Variation A");
}
Getting a flag's value
There are four methods to get a flag's value: GetStringValueAsync
, GetBooleanValueAsync
, GetIntegerValueAsync
, and GetDoubleValueAsync
. Those four methods are guaranteed to return the expected type. If the flag's value is not of the expected type, the method will return the default value you provide.
await client.GetStringValueAsync("feat", "foo");
await client.GetBooleanValueAsync("feat", true);
await client.GetIntegerValueAsync("feat", 42);
await client.GetDoubleValueAsync("feat", 3.14);
Additionally, you can use the GetObjectValueAsync
method to get the flag's value regardless of its type (including arrays and objects):
var value = await client.GetObjectValueAsync("feat", new Value("default value"));
The returned Value
class has type check methods (IsString
, IsBoolean
...) and conversion methods (AsList
, AsObject
...) to help you work with the value.
Evaluation context
When evaluating a flag you can create a context using the builder and pass it to the evaluation method:
var context = EvaluationContext.Builder()
.Set("userId", "foo")
.Set("email", "foo@gmail.com")
.Build()
await client.GetBooleanValueAsync("feat", true, context);
You can also specify the context directly on the client or globally for all evaluations:
// For this client only
client.SetContext(context);
// Globally
Api.Instance.SetContext(context);
The contexts will then be merged with the context you pass to the evaluation method.
Reporting
By default, the SDK sends flag evaluation data to Tggl to give you precious insights on how you are actually using your flags in production and to help you manage flags lifecycles better.
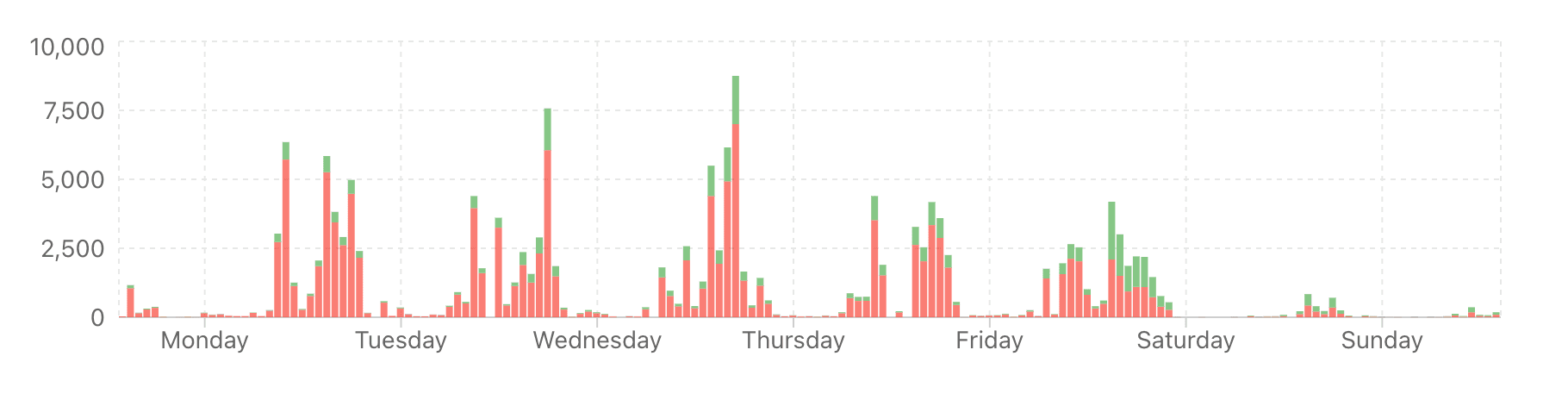
The SDK sends at most one HTTP request every 5 seconds to Tggl. Additionally, you can identify the client making the request by giving it an app name. You will be able to retrieve that name on the Tggl dashboard:
await Api.Instance.SetProviderAsync(new TgglProvider("API_KEY", new TgglClient.Options
{
Reporting = new TgglReporting.Options
{
App = "My App:2.16.3"
},
}));
You can also disable reporting entirely:
// Disable reporting when you instantiate the client
await Api.Instance.SetProviderAsync(new TgglProvider("API_KEY", new TgglClient.Options
{
Reporting = false,
}));
Using the Proxy
By default, the SDK talks directly to the Tggl API. If you are using the Tggl Proxy, you can specify the proxy URL when instantiating the client:
await Api.Instance.SetProviderAsync(new TgglProvider("API_KEY", new TgglClient.Options
{
BaseUrl = "http://your-proxy-domain.com"
}));
The /flags
and /report
path will be appended to the BaseUrl
and both flags evaluation and reporting will go through the proxy. If your proxy is configured with custom paths, you can specify them:
await Api.Instance.SetProviderAsync(new TgglProvider("API_KEY", new TgglClient.Options
{
Url = "http://your-proxy-domain.com/custom-flags",
Reporting = new TgglReporting.Options
{
Url = "http://your-proxy-domain.com/custom-report"
},
}));
Evaluate flags locally
It is possible to evaluate flags locally on the server but not recommended unless you have performance issues evaluating flags at a high frequency. Evaluating flags locally forces you to maintain the copy of flags configuration up to date and might be a source of issues.
Make sure to add the right keys to your context to be perfectly consistent with the Tggl API.
await Api.Instance.SetProviderAsync(new TggllocalProvider("YOUR_SERVER_API_KEY"));
The config will be fetched every 5 seconds by default. You can change this interval by passing a PollingInterval
parameter to the constructor.
await Api.Instance.SetProviderAsync(new TggllocalProvider("API_KEY", new TgglLocalClient.Options
{
PollingInterval = 10_000
}));
Going further
Read the official OpenFeature SDK documentation for more information on how to use the SDK.
You can also have a look at Tggl's .NET SDK documentation that is used under the hood by the TgglProvider
.