Webhooks
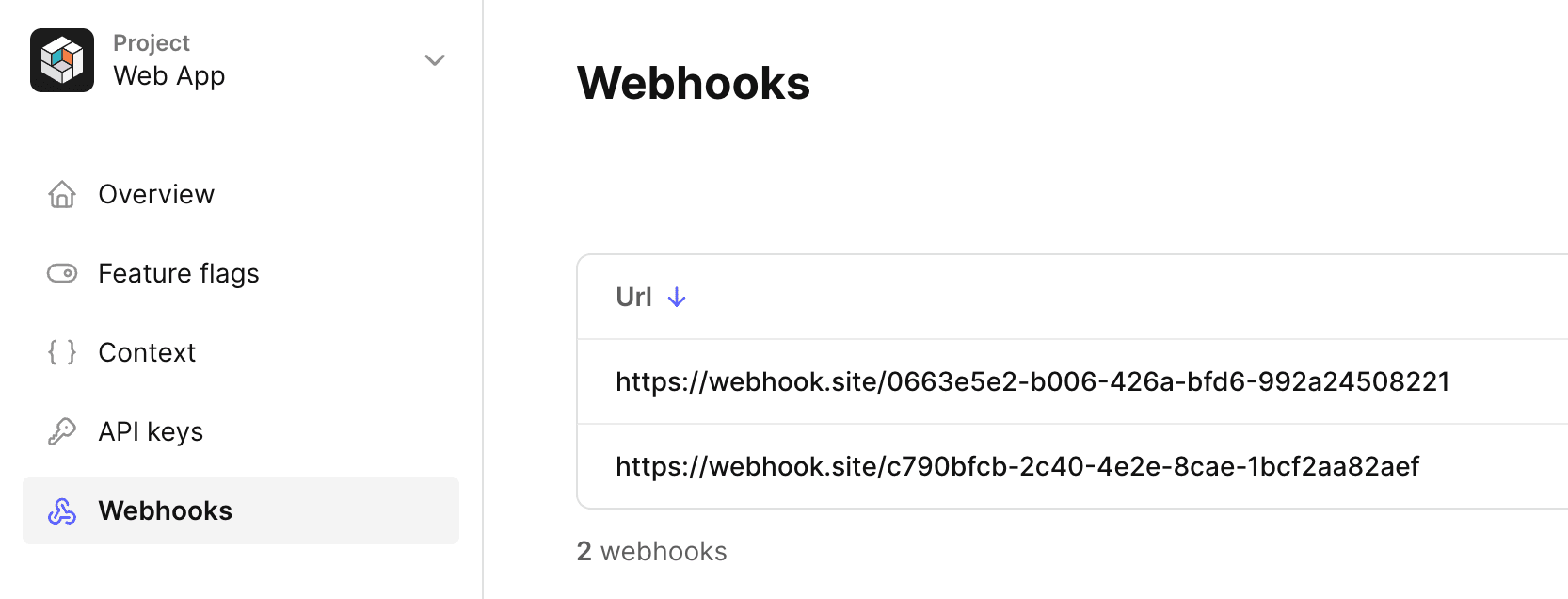
You can register webhooks on Tggl to call endpoints of your choice when events happen on Tggl. You can customize the URL and headers of the request.
Webhooks can be useful for many use-cases:
- Monitor changes on flags and display a vertical indicator on your charts to correlate metric changes with flags updates.
- Maintain a cache of you flags configuration up-to-date to evaluate flags locally without doing any API calls.
- Send a notification to your team when a flag is updated or when a change request is created.
In all cases it is up to you to implement the logic on your side.
When are webhooks called?
All webhooks of your organization are called when any event occurs.
You can use the content of the request's body to filter the events you are interested in. You can also filter events for specific projects by checking the projectSlug
key.
Authentication
You must use a header to authenticate the request. Most of the time you will
probably want to use an Authorization
header with a Bearer
:
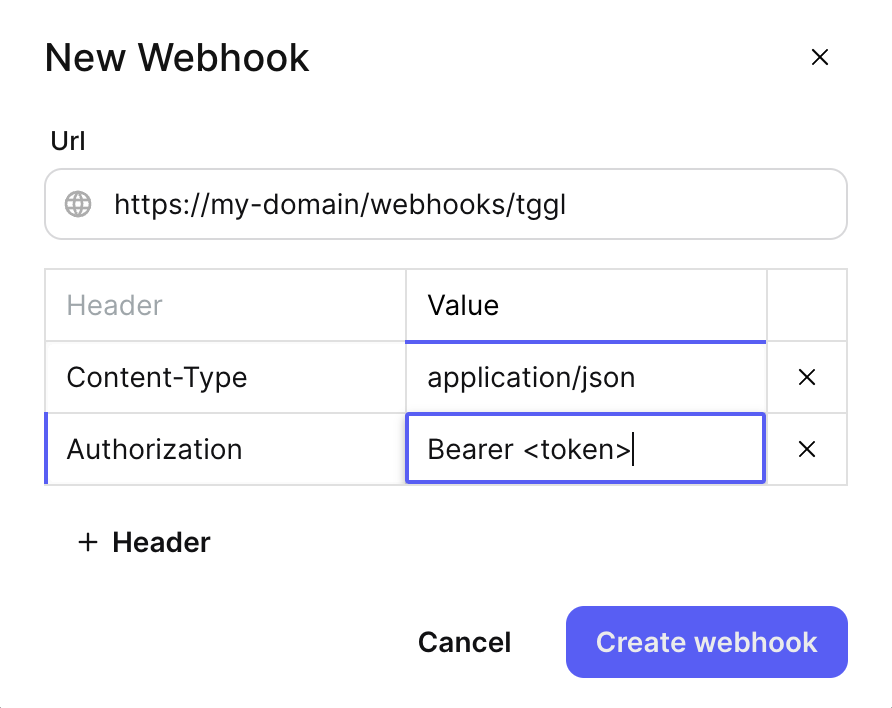
Debugging
You can debug your webhooks by sending a test request to your endpoint and displaying the list of calls. For each call you can inspect the request and the response.
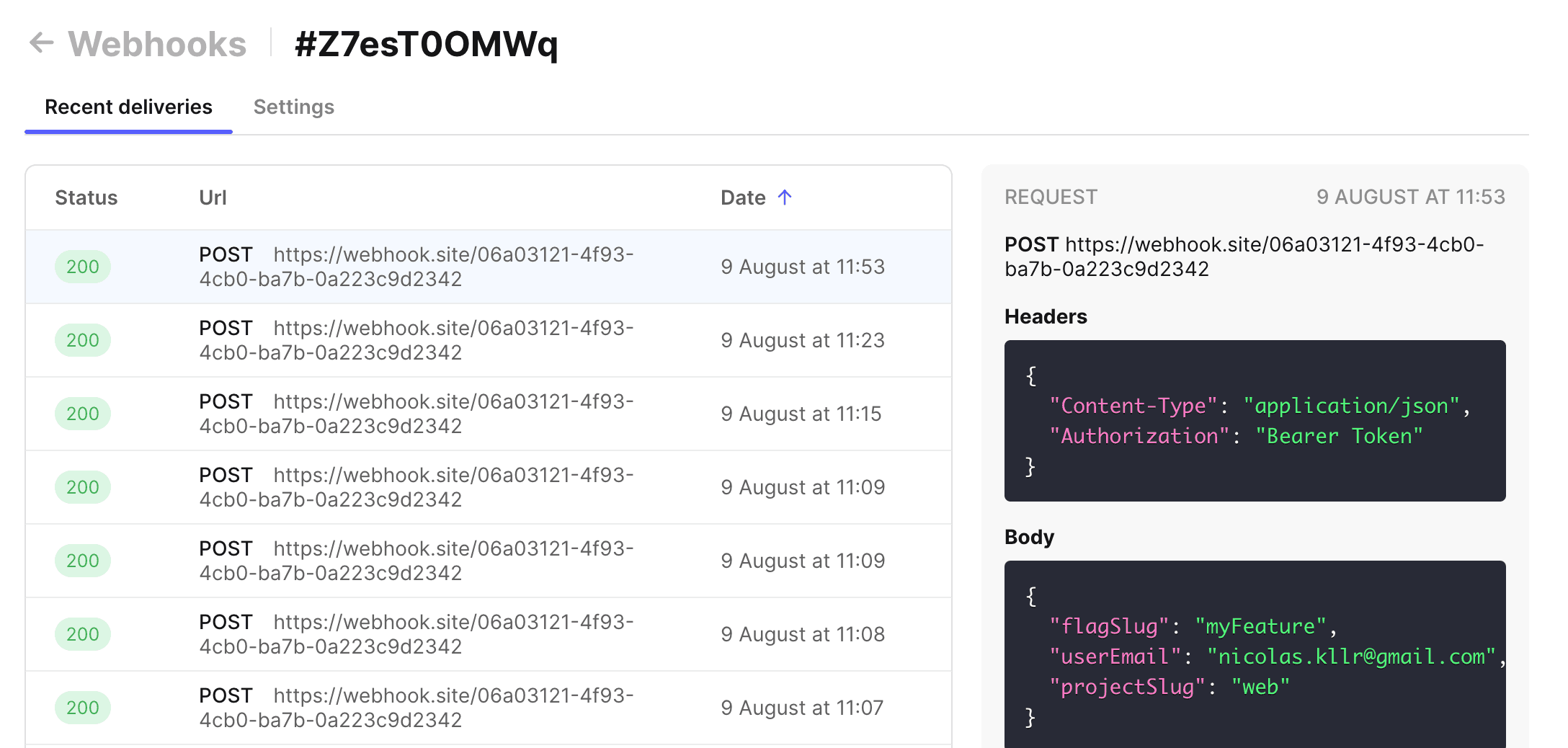
Request body
The request is always a POST
to the endpoint you specified with the following
body format:
{
"eventType": "FLAG_CHANGED",
"flagSlug": "my_feature",
"flagId": "abc123",
"logId": "def456",
"url": "https://app.tggl.io/project/android/feature-flags/abc123/history/def456",
"userEmail": "john.doe@gmail.com",
"projectSlug": "my_project",
"codeLikeBefore": "IF ... THEN ... ELSE ...",
"codeLikeAfter": "IF ... THEN ... ELSE ...",
"changes": [
{ "__typename": "FlagConditionCreated" /*...*/ },
{ "__typename": "FlagDescriptionChanged" /*...*/ }
]
}
flagSlug
The flagSlug
key is the slug of the flag that was updated, you can find it
on the app:
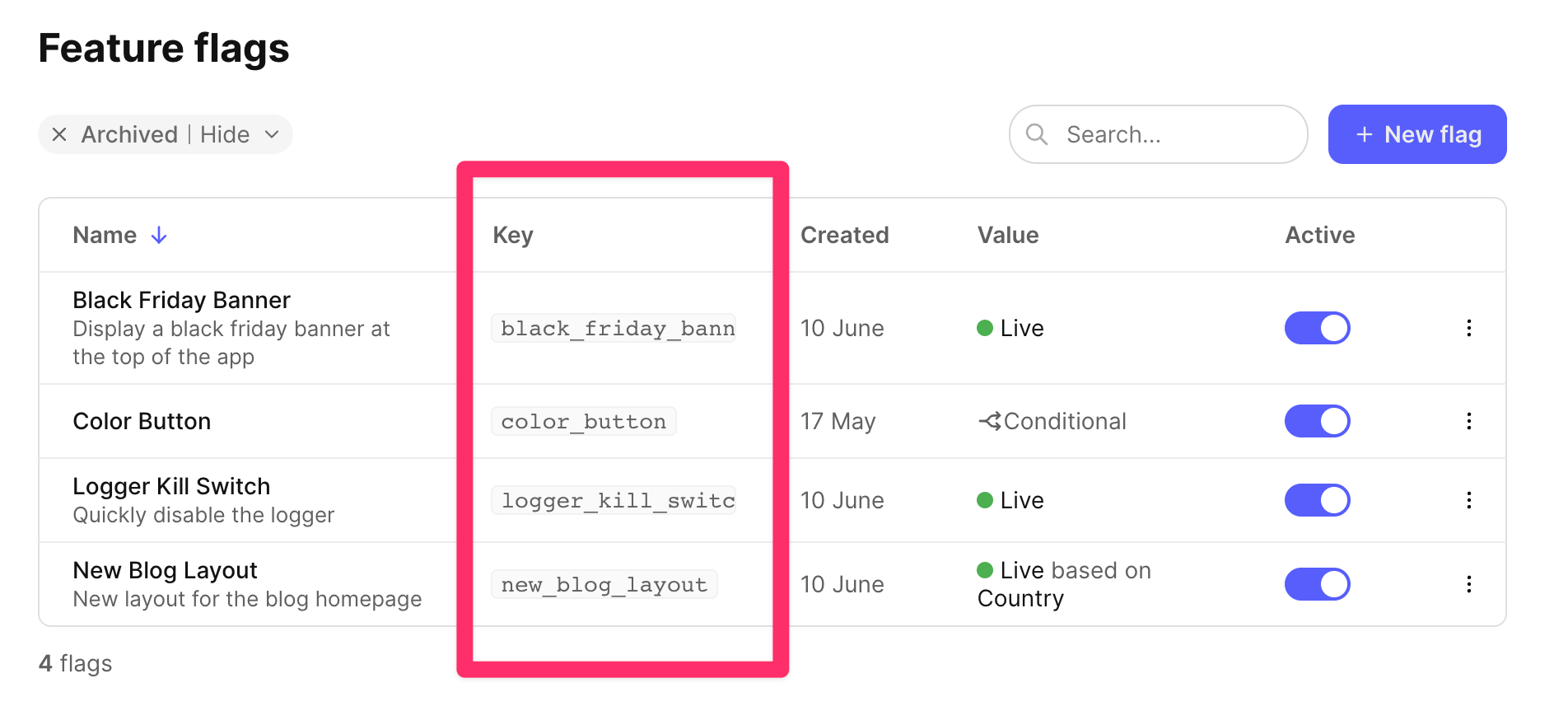
projectSlug
The projectSlug
key is the slug of the project of the flag and can also be
found on the app:
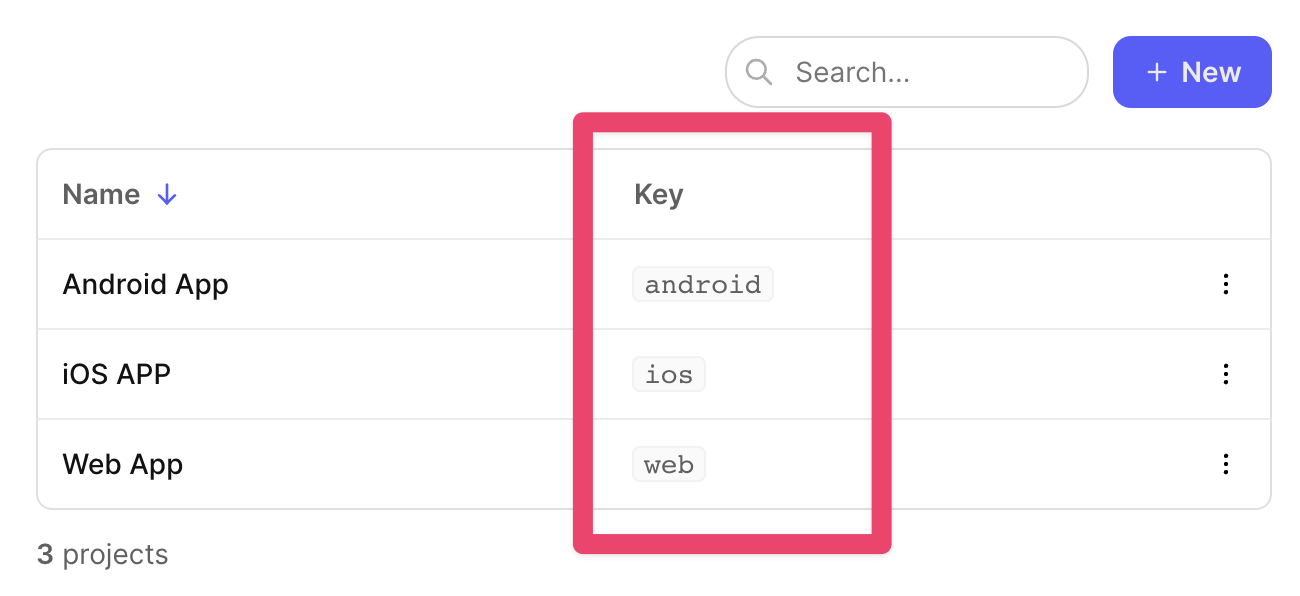
codeLikeBefore
and codeLikeAfter
The codeLikeBefore
and codeLikeAfter
keys are a string representation of the flag's conditions before and after the change. You can use them to display it directly to humans and even display a diff of the change. The code-like representation looks like this:
IF
userId IS ONE OF [
"foo",
"bar",
]
THEN
Live (value: true)
ELSE IF
environment == "production"
AND 20% OF userId (0% TO 20%)
AND timestamp >= "2023-12-11T00:00"
THEN
Live (value: true)
ELSE
Off (value: false)
Note that the code-like representation should not be parsed, it is only meant to be displayed to humans.
changes
The changes
key is an array of FlagChange
that were applied to the flag. Each change is an object with a __typename
key that can be used to know which fields are available on the change object. See the schema for more details.