Local evaluation
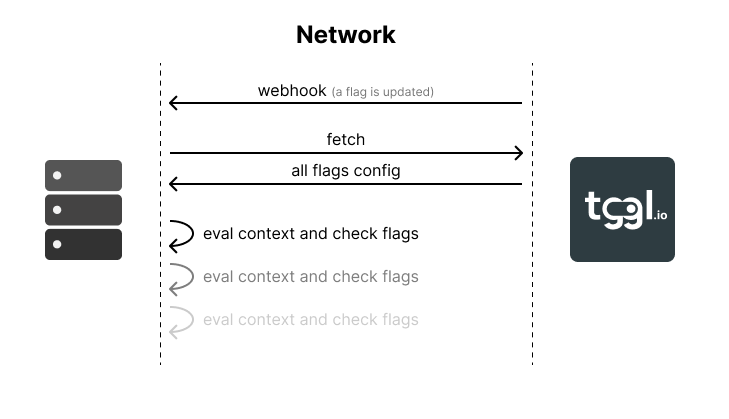
You should not evaluate flags locally on client-side applications to prevent exposing sensitive information to the client.
It is recommended to evaluate flags via the API unless you have performance issues or if you need to split traffic on the edge without doing an API call.
Evaluating flags locally will let you evaluate millions of contexts extremely efficiently without making any API calls.
Using an SDK
This can be done easily in all server-side SDKs (see documentation):
import { TgglLocalClient } from 'tggl-client'
const client = new TgglLocalClient('YOUR_SERVER_API_KEY')
await client.fetchConfig()
if (client.get({ userId: 'foo' }, 'my-feature', false)) {
//...
}
Here the fetchConfig
method is fetching the config from the API, and get
is evaluating a context against a flag without making any API call.
Using the API directly
If your language does not have an SDK, or you prefer to use the API directly, you can make the HTTP call yourself (see documentation).
curl 'https://api.tggl.io/config' \
-H 'x-tggl-api-key: <server api key>'
All SDKs are simply doing this call under the hood, and then caching the result in memory.
Keeping flags definition up to date
You have two solutions to maintain the cache of the configuration up to date:
- Do some polling and re-fetch the config at a regular intervals, this is usually a simple configuration in the SDK
- Use a webhook to update the cache when a flag is updated
It is recommended to use both a webhook for fast updates, and polling with a large interval as a safety net.
Important differences with the API
When evaluating flags via the API a few keys are automatically added to the context. When evaluating flags locally it is your responsibility to pass those keys to the context, otherwise any condition that is based on those keys will fail.
If you do not manually pass those keys to the context you might have discrepancies between evaluating flags locally and via the API.
Here is the exhaustive list of keys that are automatically added by Tggl when evaluating flags via the API:
timestamp
: the current timestampip
: the IP address that made the HTTP requestreferer
: the value of thereferer
HTTP header
Caching and resiliency
An important aspect of resilience is to cache the configuration in a storage and to have a fallback mechanism in case the API is down and you need to restart your sever.
You do not have to implement it yourself and can simply use the Tggl Proxy that mimics 1-for-1 the API but runs on your infrastructure and managees caching and batching.
const client = new TgglLocalClient('YOUR_API_KEY', {
baseUrl: 'http://your-proxy-domain.com'
})