Remote evaluation
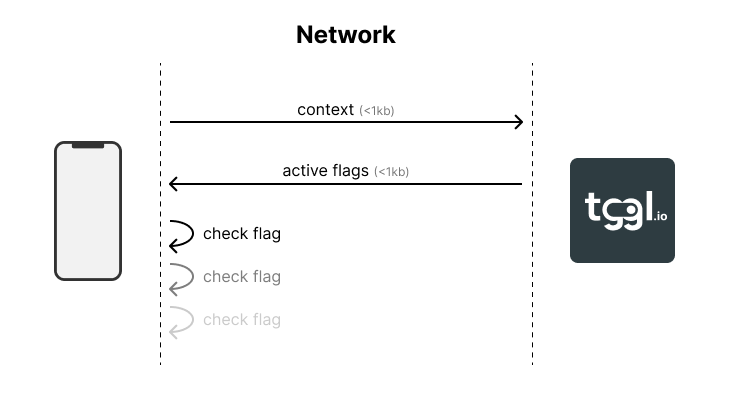
For client-side applications, the recommended way to evaluate flags is to use the API. This way you do not expose any sensitive information to the client, and you have a consistent evaluation strategy across all environments without re-implementing the logic.
You only need to perform a single API call when the context changes, typically when you load the app or when the user logs in. From that single call you receive all flags at once and can simply look up the list of flags to see if a particular feature should be active or not.
The API can also be used server-side to evaluate flags for a large number of contexts at once to avoid un-necessary round-trips.
Using an SDK
This pattern can be done easily in all SDKs (see documentation):
import { TgglClient } from 'tggl-client'
const client = new TgglClient('YOUR_API_KEY')
const flags = await client.evalContext(context)
if (flags.get('my-feature', false)) {
// ...
}
Here the evalContext
method is making the API call under the hood, returning the result in a class that has a get
method that does not make any API call.
Some front-end SDKs "hide" this logic a bit more, storing the response in a global state, but the logic is exactly the same: a single API call is made when the context changes.
Using the API directly
If your language does not have an SDK, or you prefer to use the API directly, you can make the HTTP call yourself (see documentation).
curl 'https://api.tggl.io/flags' \
-H 'Content-Type: application/json' \
-H 'x-tggl-api-key: <api key>' \
--data-raw '{"userId":"foo","email":"foo@gmail.com"}'
All SDKs simply wrap this call and provide a more convenient interface.
Re-evaluating flags when they change
Flag changes are not pushed to the client, changing a flag will not automatically update your app in real time. While most app do not need this feature, if that is something you need for your application you have two options:
- Poll the API at regular intervals to check if flags have changed. Most SDKs support a polling interval option out of the box. Note that polling will increase the number of API calls you make, so you should only enable it if you need it.
- Use a webhook to notify your back-end when flags are changed. It is then up to you to notify your clients using websockets or any other real-time method.
Latency and redundancy
The API is designed to be fast and reliable, but it is still a network call that can be slow or fail. This is why we recommend to add a Tggl Proxy that runs on your infrastructure in a simple docker container.
The proxy will mick 1-for-1 the Tggl API, and will manage caching and batching for you. This way you can have a very low latency and high availability, even if the Tggl API is down.
The only thing you have to do is run the container on your infrastructure and point your SDKs to it instead of the Tggl API.
const client = new TgglClient('YOUR_API_KEY', {
baseUrl: 'http://your-proxy-domain.com'
})